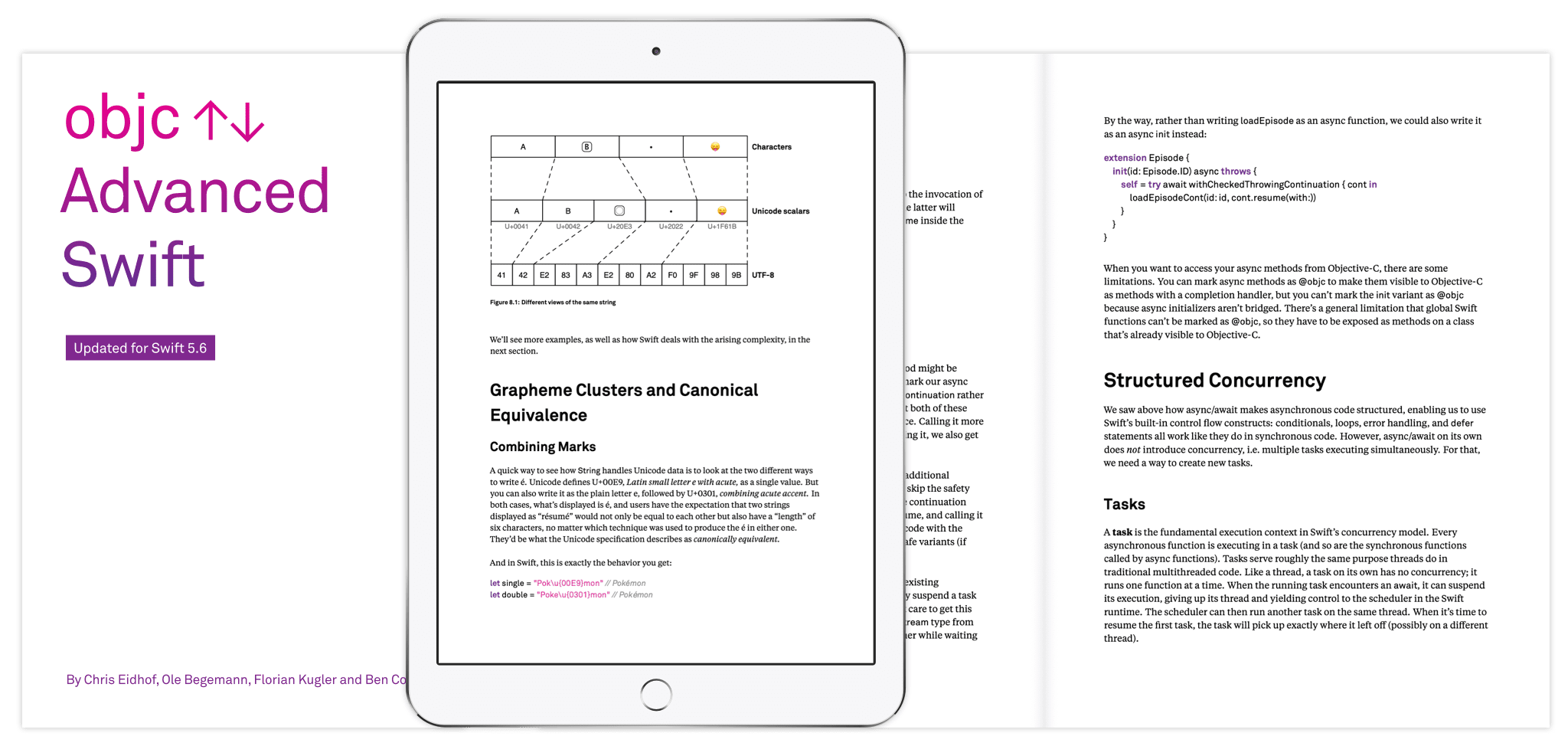
Updated for Swift 5.6
Advanced Swift
A deep dive into Swift’s features, from low-level programming to high-level abstractions.
by Chris Eidhof, Ole Begemann, Florian Kugler, and Ben Cohen
In this book, we'll write about advanced concepts in Swift programming. If you have read the Swift Programming Guide, and want to explore more, this book is for you.
Swift is a great language for systems programming, but also lends itself for very high-level programming. We'll explore both high-level topics (for example, programming with generics and protocols), as well as low-level topics (for example, wrapping a C library and string internals).
Book Contents
-
Concurrency
How to use Swift's structured concurrency system.
-
Collections
How to use Swift's built-in collection types and how to build your own.
-
Optionals
Improve the safety and clarity of your code.
-
Generics
Write functions and methods that work on multiple types.
-
Protocols
Use protocols to simplify your code.
-
Strings
How to use strings and how they work with unicode.
-
Enums
Use enums to model your data precisely.
-
Mutability
Use Swift's powerful features to control mutability.
-
Memory Management
Learn how value types and reference types interact.
-
Error Handling
Understand Swift's built-in error handling.
-
Interoperability
Wrap calls to C libraries and present them in Swift using a natural API.
Video Episodes (Full Table of Contents)
-
C Interoperability 1h12min
Advanced C interop when wrapping the Cairo graphics library.
-
String Parsing 1h
We extend the CSV parser from the book to be a maintainable, high-performance parser.
-
Collection Protocols 58min
We show how to create a custom sorted array data structure and work with collection protocols.
-
Functions 36min
We show how to leverage first-class functions and generics to build a validation library.
-
Encoding & Decoding Graphs 46min
We show how to represent cyclical data structures that work with Codable.
Bundles
Save on each additional book
About the Authors
-
Chris Eidhof
Chris is one of the objc.io co-founders, and host of Swift Talk. He also co-authored the Functional Swift, Advanced Swift, and Thinking in SwiftUI books. Before, he wrote apps such as Deckset.
-
Ole Begemann
Ole is a freelance app developer, writer, and occasional technical editor. He's been writing about Swift on his blog since the language was first released. For some strange reason, he finds string handling and Unicode particularly fascinating
-
Florian Kugler
Florian is one of the objc.io co-founders. He worked on Mac Apps like Deckset, co-authored the Thinking in SwiftUI book amongst others, and hosts the weekly Swift Talk video series. Recently, he also started running Anton Reisemobile part time, a workshop for fine campervan conversions.
-
Ben Cohen
Ben Cohen is a member of the Swift core team. He started writing about Swift during the first beta at airspeedvelocity.net, and currently works on the Swift standard library.
FAQ
-
Is the book using Swift 5.6?
Yes, it's been fully rewritten for Swift 5.6.
-
Will you update the book?
Yes. For more information on our policy, see book updates.
-
Do you offer student discounts?
Yes, please email us and let us know what and where you're studying.
-
Do you provide training?
Yes, we provide workshops and training. If you're interested, email us.
-
Can I get a “reverse charge” invoice without VAT?
Just click the "Generate Invoice" link in the receipt email and fill out your company data to get a VAT refund.
Updates
-
March 2022
Updated for Swift 5.6
-
May 2019
Updated for Swift 5
-
November 2017
Updated for Swift 4
-
October 2016
Updated for Swift 3
-
March 2016
Initial relase, in Swift 2.2
-
July 2015
Early Access, in Swift 2.0